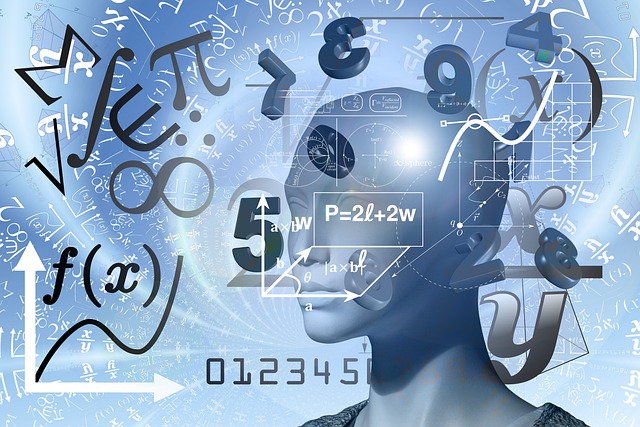
In today’s fast-paced digital world, automation has become a cornerstone of efficiency. Among the tools driving this trend are bots, especially Telegram bots. Telegram, known for its security and flexibility, provides an excellent platform for integrating bots to perform various tasks, from customer support to content delivery. Building a Telegram bot might seem daunting at first, but with the right guidance, even beginners can create functional and versatile bots. Here’s a comprehensive guide to help you get started.
What Is a Telegram Bot?
A Telegram bot is an automated program that interacts with users, groups, or channels on Telegram. It can perform specific tasks such as sending notifications, managing groups, or even playing games. These bots operate via the Telegram Bot API, which allows developers to connect their software to Telegram’s platform.
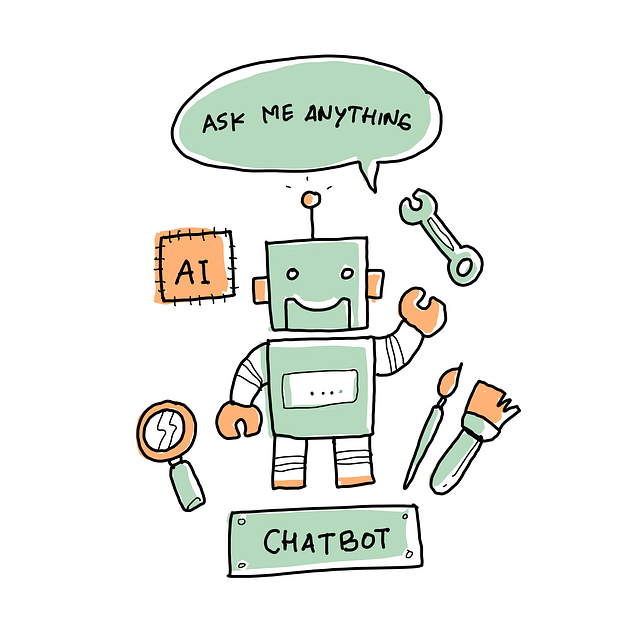
Why Should You Build a Telegram Bot?
- Automate Repetitive Tasks: Bots can handle repetitive tasks like sending reminders or managing FAQs, saving time.
- Enhanced User Engagement: Whether for business or personal use, bots improve user interaction through features like polls, quizzes, or personalized messages.
- Integration with Services: You can integrate Telegram bots with APIs, databases, or other platforms to offer seamless services.
- Cost-Efficiency: Bots eliminate the need for manual intervention, reducing costs in customer support or operational tasks.
What You’ll Need to Get Started
Before you begin, ensure you have the following:
- Telegram Account: You need a Telegram account to interact with BotFather and test your bot.
- Basic Programming Knowledge: Familiarity with Python, JavaScript, or PHP will be helpful.
- Hosting Service: Platforms like Heroku, AWS, or a personal server are needed to deploy your bot.
- Telegram Bot API Key: This is provided by Telegram’s BotFather and is crucial for connecting to Telegram servers.
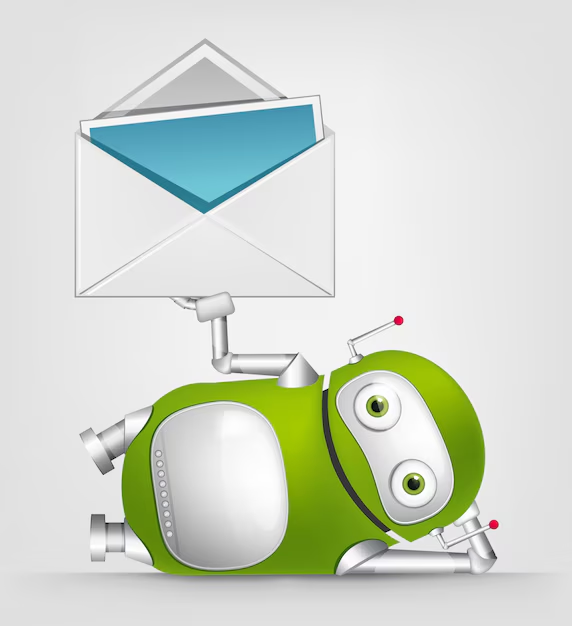
Step 1: Setting Up the Bot
Create a Bot Using BotFather
- Open Telegram and search for “BotFather.”
- Start a chat with BotFather and send the command
/newbot
. - Follow the prompts to name your bot and assign it a unique username.
- Once the bot is created, BotFather will provide an API token. Keep this token secure, as it’s required for all interactions with Telegram servers.
Test Your Bot
Copy the bot’s username and send it a message to verify that it responds. This basic interaction confirms that your bot is active.
Step 2: Setting Up Your Development Environment
Choose a Programming Language
While there are many languages available, Python is the most popular for Telegram bot development, thanks to its simplicity and robust libraries like python-telegram-bot
.
Install Required Libraries
For Python users, install the necessary libraries using pip:
pip install python-telegram-bot
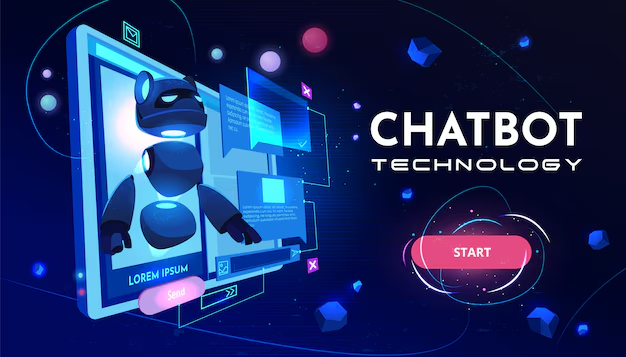
Create a Skeleton Script
Here’s a basic structure to get started:
from telegram import Update
from telegram.ext import Updater, CommandHandler, CallbackContext
def start(update: Update, context: CallbackContext) -> None:
update.message.reply_text('Hello! I am your bot.')
def main():
updater = Updater("YOUR_API_KEY_HERE")
dispatcher = updater.dispatcher
dispatcher.add_handler(CommandHandler("start", start))
updater.start_polling()
updater.idle()
if __name__ == '__main__':
main()
Replace YOUR_API_KEY_HERE
with the token you received from BotFather.
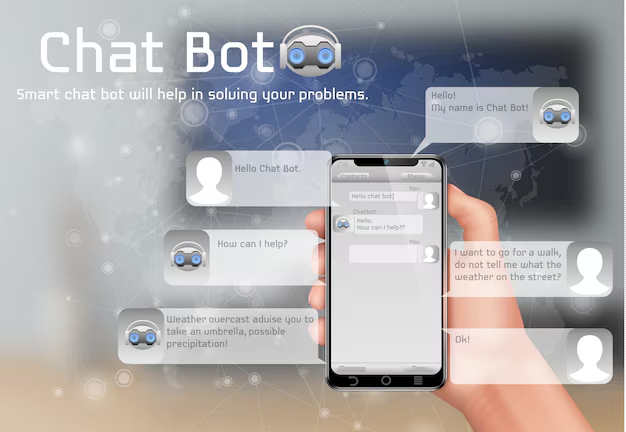
Step 3: Adding Functionality
Command Handlers
Bots respond to specific commands, such as /help
or /info
. Define handlers for each command to control the bot’s responses.
def help(update: Update, context: CallbackContext) -> None:
update.message.reply_text('List of commands:\n/start - Start the bot\n/help - Get help')
Custom Features
Add more interactive features like message echoing or integration with external APIs:
def echo(update: Update, context: CallbackContext) -> None:
update.message.reply_text(update.message.text)
Register the handler:
dispatcher.add_handler(MessageHandler(Filters.text & ~Filters.command, echo))
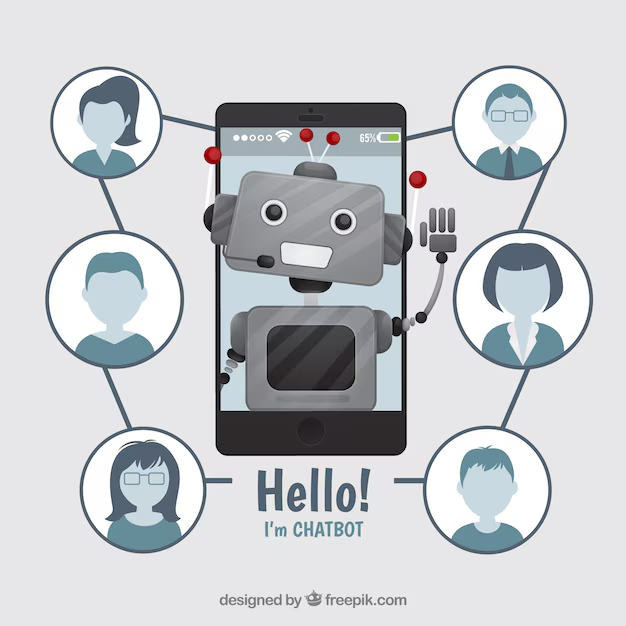
Step 4: Hosting Your Bot
To keep your bot running 24/7, deploy it on a hosting platform.
Using Heroku
- Install the Heroku CLI and create an account.
- Create a
requirements.txt
file listing the libraries your bot uses. - Define a
Procfile
to specify the command to run your bot. For example:worker: python your_script.py
- Push your code to Heroku, and start the bot.
Step 5: Enhancing the Bot with Advanced Features
Connect to External APIs
Bots become more functional when integrated with APIs. For instance, you can fetch weather data or stock prices.
Example: Fetching weather data using OpenWeather API:
import requests
def weather(update: Update, context: CallbackContext) -> None:
city = update.message.text.split(' ')[1]
api_url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid=YOUR_API_KEY"
response = requests.get(api_url).json()
if response.get("weather"):
weather_desc = response["weather"][0]["description"]
update.message.reply_text(f"The weather in {city} is {weather_desc}.")
else:
update.message.reply_text("City not found.")
Register the command:
dispatcher.add_handler(CommandHandler("weather", weather))
Database Integration
For bots that need to store user data, integrate with a database like SQLite or Firebase.
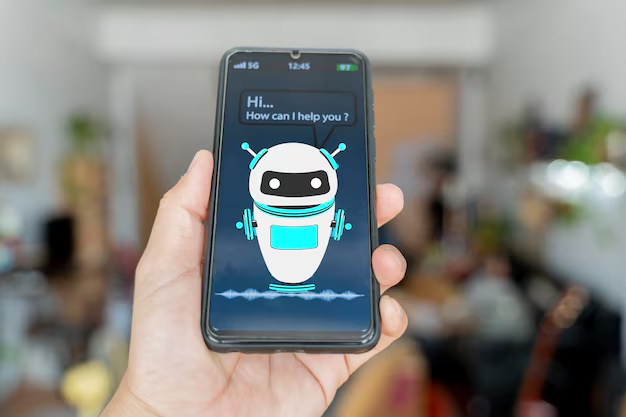
Step 6: Secure Your Bot
- Protect the API Key: Never share your bot’s API key publicly.
- Set Permissions: Restrict access to sensitive commands or features.
- Monitor Usage: Use logging to track errors and user interactions.
Conclusion
Building a Telegram bot is a rewarding experience that opens doors to numerous possibilities. Whether you’re automating tasks, engaging users, or exploring API integrations, bots are a powerful tool in the digital age. With the steps outlined above, you can create a functional bot and continue to refine its capabilities as you grow more comfortable with the development process.
FAQ
- Can I build a Telegram bot without coding?
Yes, platforms like ManyChat and Chatfuel allow you to create bots without programming knowledge. - Is hosting a bot free?
Many platforms offer free tiers, but advanced bots may require paid hosting services. - How do I monetize my bot?
You can charge users for premium features, integrate ads, or offer subscription-based services. - Can I use any programming language to build a Telegram bot?
Yes, Telegram’s Bot API supports multiple languages like Python, JavaScript, and PHP. - Are Telegram bots safe?
Yes, as long as you follow best practices like securing API keys and using encrypted connections.